ChatGPT-Like App : In this blog, we will walk through a simple example of creating a local ChatGPT-Like App that simulates a ChatGPT-like experience using HTML and JavaScript. We’ll break down the code and explain how it all fits together to build a functional chat interface.
Register OpenAI models
To start with the gen AI model, you need to set up a model at your local or you can use a free account for your setup by creating an account below.
https://aistudio.google.com/app/apikey
HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ChatGPT UI</title>
<style>
/* Styles go here */
</style>
</head>
<body>
<div class="chat-container">
<div class="chat-header">
<h1>ChatGPT</h1>
</div>
<div class="chat-messages" id="chatMessages">
<!-- Messages will be appended here -->
</div>
<div class="chat-input">
<input type="text" id="userInput" placeholder="Type your message here..." />
<button onclick="sendMessage()">Send</button>
</div>
</div>
<script>
// JavaScript goes here
</script>
</body>
</html>
CSS Styling
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
font-family: Arial, sans-serif;
background-color: #f4f4f9;
}
.chat-container {
width: 400px;
background-color: #ffffff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
border-radius: 10px;
overflow: hidden;
}
.chat-header {
background-color: #2d2d2d;
color: white;
padding: 10px;
text-align: center;
}
.chat-messages {
padding: 10px;
height: 500px;
overflow-y: auto;
border-bottom: 1px solid #ddd;
}
.chat-input {
display: flex;
padding: 10px;
}
.chat-input input {
flex: 1;
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
.chat-input button {
padding: 10px 20px;
background-color: #2d2d2d;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
margin-left: 10px;
}
.chat-input button:hover {
background-color: #1a1a1a;
}
.message {
margin-bottom: 10px;
}
.user-message {
text-align: right;
}
.bot-message {
text-align: left;
background-color: #f1f1f1;
padding: 10px;
border-radius: 5px;
}
Read Also : Guide 101 : How to Write Effective Prompts
1. Body Styling
The body
is styled to center the chat container both horizontally and vertically. The background color and font are set to provide a clean and modern look.
2. Chat Container
The .chat-container
is styled with a fixed width, background color, box shadow for a subtle 3D effect, and rounded corners to enhance its appearance.
3. Header and Messages
The .chat-header
sets the background color and text color for the header. The .chat-messages
area is styled to handle scrolling and has a border to separate it from the input area.
4. Input and Button
The .chat-input
section styles the text input and button to ensure they are user-friendly. The button changes color on hover for better interaction feedback.
JavaScript Functionality
const chatMessages = document.getElementById('chatMessages');
const API_KEY = 'YOUR_API_KEY_HERE';
const API_URL = 'https://api.together.xyz/v1/chat/completions';
const userInput = document.getElementById('userInput');
userInput.addEventListener('keyup', function(event) {
if (event.key === 'Enter') {
sendMessage();
}
});
async function sendMessage() {
const input = document.getElementById('userInput');
const userMessage = input.value.trim();
if (userMessage) {
appendMessage(userMessage, 'user-message');
input.value = '';
const response = await fetch(API_URL, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`
},
body: JSON.stringify({
"model": "meta-llama/Meta-Llama-3.1-8B-Instruct-Turbo",
"messages": [
{
"role": "user",
"content": userMessage
}
]
})
});
const data = await response.json();
appendMessage(data.choices[0].message.content, 'bot-message');
}
}
function appendMessage(message, className) {
const messageElement = document.createElement('div');
messageElement.classList.add('message', className);
messageElement.textContent = message;
chatMessages.appendChild(messageElement);
chatMessages.scrollTop = chatMessages.scrollHeight;
}
Read Also : The Art of Prompt Engineering: Mastering the Language of Large Language Models (LLMs)
1. Event Listener for Enter Key
The userInput
element listens for the Enter key to trigger the sendMessage
function, allowing for easy message submission.
2. Send Message Function
The sendMessage
function captures the user’s input, sends it to the API, and appends both the user’s message and the bot’s response to the chat window.
3. Append Message Function
The appendMessage
function creates a new message element, styles it according to whether it’s from the user or the bot, and appends it to the chat window. It also scrolls to the bottom to show the latest message.
Complete Code – ChatGPT-Like App
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ChatGPT UI</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
font-family: Arial, sans-serif;
background-color: #f4f4f9;
}
.chat-container {
width: 400px;
background-color: #ffffff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
border-radius: 10px;
overflow: hidden;
}
.chat-header {
background-color: #2d2d2d;
color: white;
padding: 10px;
text-align: center;
}
.chat-messages {
padding: 10px;
height: 500px;
overflow-y: auto;
border-bottom: 1px solid #ddd;
}
.chat-input {
display: flex;
padding: 10px;
}
.chat-input input {
flex: 1;
padding: 10px;
border: 1px solid #ddd;
border-radius: 5px;
}
.chat-input button {
padding: 10px 20px;
background-color: #2d2d2d;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
margin-left: 10px;
}
.chat-input button:hover {
background-color: #2d2d2d;
}
.message {
margin-bottom: 10px;
}
.user-message {
text-align: right;
}
.bot-message {
text-align: left;
background-color: #f1f1f1;
padding: 10px;
border-radius: 5px;
}
</style>
</head>
<body>
<div class="chat-container">
<div class="chat-header">
<h1>ChatGPT</h1>
</div>
<div class="chat-messages" id="chatMessages">
<!-- Messages will be appended here -->
</div>
<div class="chat-input">
<input type="text" id="userInput" placeholder="Type your message here..." />
<button onclick="sendMessage()">Send</button>
</div>
</div>
<script>
const chatMessages = document.getElementById('chatMessages');
const API_KEY = 'YOUR_API_KEY_HERE';
const API_URL = 'https://api.together.xyz/v1/chat/completions';
const userInput = document.getElementById('userInput');
userInput.addEventListener('keyup', function(event) {
if (event.key === 'Enter') {
sendMessage();
}
});
async function sendMessage() {
const input = document.getElementById('userInput');
const userMessage = input.value.trim();
if (userMessage) {
appendMessage(userMessage, 'user-message');
input.value = '';
const response = await fetch(API_URL, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${API_KEY}`
},
body: JSON.stringify({
"model": "meta-llama/Meta-Llama-3.1-8B-Instruct-Turbo",
"messages": [
{
"role": "user",
"content": userMessage
}
]
})
});
const data = await response.json();
appendMessage(`${data.choices[0].message.content}`, 'bot-message');
}
}
function appendMessage(message, className) {
const messageElement = document.createElement('div');
messageElement.classList.add('message', className);
messageElement.textContent = message;
chatMessages.appendChild(messageElement);
chatMessages.scrollTop = chatMessages.scrollHeight;
}
</script>
</body>
</html>
Read Also: The Rise of Large Language Models: A Revolution in AI
Demo on ChatGPT-Like App
Let’s see what it looks like after completion.

Conclusion : ChatGPT-Like App
This simple HTML and JavaScript code provides a foundational chat interface similar to ChatGPT-Like App. You can build and expand upon this example by understanding and customizing HTML, CSS, and JavaScript to create a more complex and feature-rich chat application.
Video Explanation
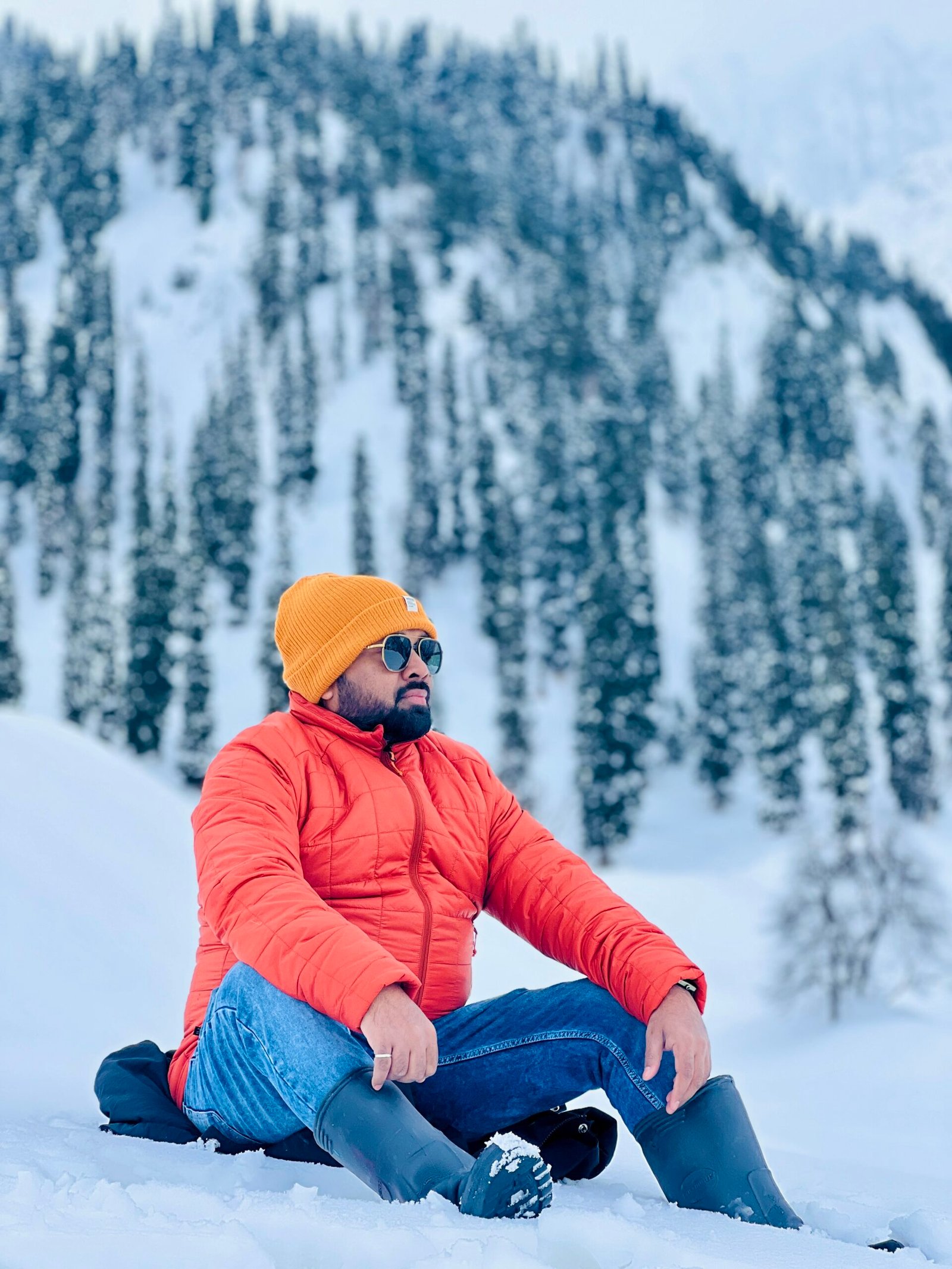
Hey Tech Enthusiasts!
I’m Avinash, a passionate tech blogger with over 13+ years of experience in the trenches of software engineering.
You could say I’ve worn many hats in my journey – from full-stack developer crafting beautiful and functional applications to Solution Architect, designing the architecture for complex systems.
Over the years, I’ve delved into a vast arsenal of languages and tools, including the Generative AI (LLMs, LLM-Proxy, Observability, Prompt Engineering), .NET family (.NET, .NET Core), PHP, Rust, Python, the JavaScript frameworks (Angular, React, Node.js), and databases like MySQL, SQL Server, MongoDB.
As the cloud revolutionized our world, I’ve become well-versed in both Azure and GCP platforms, wielding Docker for containerization and CI/CD pipelines to streamline development workflows.
Here on my blog, I aim to share the knowledge I’ve accumulated and the lessons I’ve learned along the way. Whether you’re a seasoned developer or just starting your coding adventure, I want to provide you with insightful, practical articles that tackle real-world tech challenges.
Get ready to explore the latest advancements, delve into programming concepts, and discover efficient solutions to your development dilemmas. So, buckle up, tech enthusiasts – let’s embark on this exciting journey together!